Code is copied!
Question 6
Define a class to accept values into 4x4 array and find and display the sum of each row.
Example: A[][]={1,2,3,4},{5,6,7,8},{1,3,5,7},{2,5,3,1}}
Output:
sum of row 1 =10 (1+2+3+4)
sum of row 2= 26 (5+6+7+8)
sum of row 3=16 (1+3+5+7)
sum of row 4= 11 (2+5+3+1)
Solution:
,
import java.util.Scanner;
class ArrayRowSum
{
public static void main(String[] args)
{
Scanner sc = new Scanner(System.in);
int[][] arr = new int[4][4];
System.out.println("Enter the values for a 4x4 array:");
for (int i = 0; i < 4; i++)
{
for (int j = 0; j < 4; j++)
{
arr[i][j] = sc.nextInt();
}
}
for (int i = 0; i < 4; i++)
{
int sum = 0;
for (int j = 0; j < 4; j++)
{
sum += arr[i][j];
}
System.out.println("Sum of row " + (i + 1) + " = " + sum);
}
}
}
Define a class to accept values into 4x4 array and find and display the sum of each row. Example: A[][]={1,2,3,4},{5,6,7,8},{1,3,5,7},{2,5,3,1}} Output: sum of row 1 =10 (1+2+3+4) sum of row 2= 26 (5+6+7+8) sum of row 3=16 (1+3+5+7) sum of row 4= 11 (2+5+3+1)
Solution:
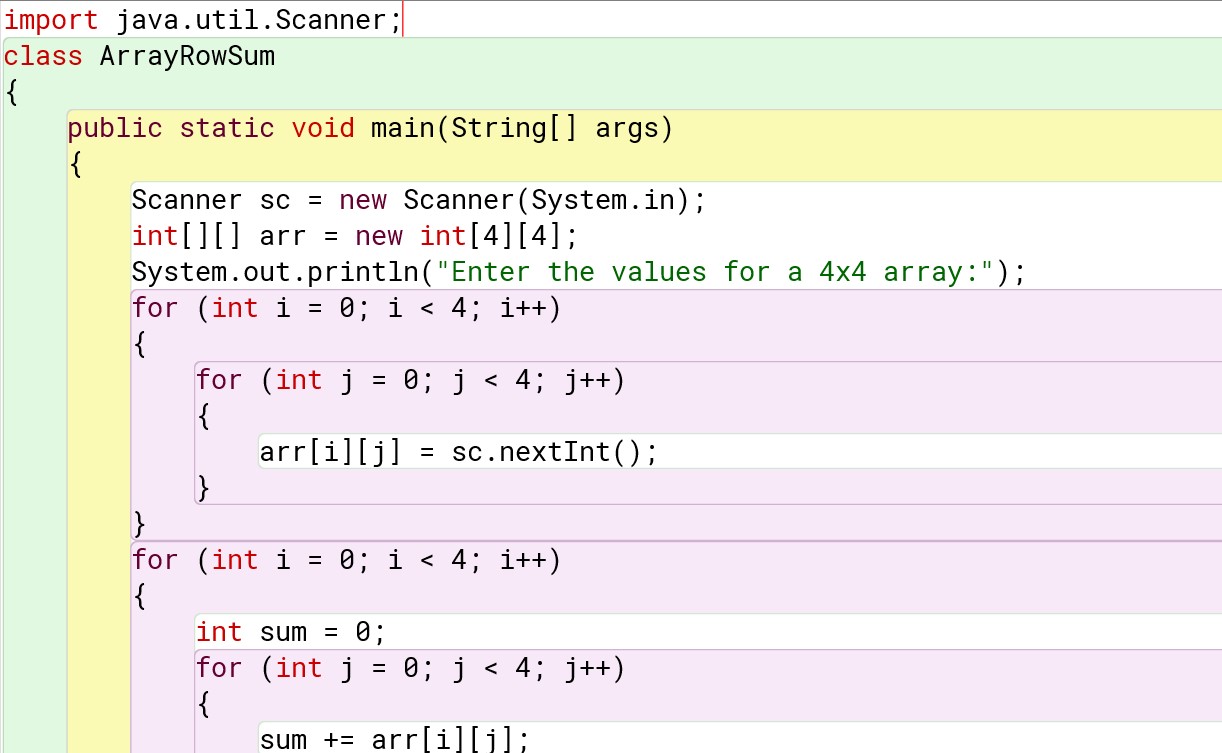

import java.util.Scanner;
class ArrayRowSum
{
public static void main(String[] args)
{
Scanner sc = new Scanner(System.in);
int[][] arr = new int[4][4];
System.out.println("Enter the values for a 4x4 array:");
for (int i = 0; i < 4; i++)
{
for (int j = 0; j < 4; j++)
{
arr[i][j] = sc.nextInt();
}
}
for (int i = 0; i < 4; i++)
{
int sum = 0;
for (int j = 0; j < 4; j++)
{
sum += arr[i][j];
}
System.out.println("Sum of row " + (i + 1) + " = " + sum);
}
}
}